What's it like to call your API? Developers want to use your API to accomplish something, and you want to make it as easy as possible for them to incorporate your service. To allow developers to call your API directly from any technology, your docs provide DIY instructions. To go a step further and give developers additional conveniences and tooling, you can provide Software Development Kits (SDKs). SDKs include code to call your API so that developers don't have to write it themselves from scratch.
While calling an API directly vs. using an SDK does not change the ultimate functionality of the API, it can dramatically affect the developer experience. In most cases, one method will be preferred over the other, varying according to each developer’s needs, preferences, and skills. An SDK offers simplicity and speedier development but applies only to a particular development framework. SDKs require resources to develop and maintain. Direct calls offer platform flexibility and are available to all developers, but are harder to use without expertise.
In this article, we'll be looking at why your developer experience may focus on direct API calls, SDKs, or both.
Direct API Calls: The Basics
For communicating with a service, sending API calls directly to URL endpoints is the simplest way. Of course, when we say simplest, we mean in terms of program complexity, not ease of use. When using an API without an SDK, you make calls to its URL endpoints directly using standard internet protocol commands for transferring packets of information over the network. There's no abstraction between you and the service, so every parameter must be written into the call. It must also conform to a standard format.
An example of how to construct a direct API call using Python is shown in the code snippet below. Notice the URL for the API endpoint is specified explicitly, alongside required header parameters that describe the request. This information is bundled and transmitted using the requests
library, which then receives any response data returned by the API and stores it in the resp
variable. Note that the cost of deserialization is added here for the API call.
import requests
from requests.structures import CaseInsensitiveDict
url = "https://connect.squareup.com/v2/payments"
headers = CaseInsensitiveDict()
headers["Square-Version"] = "2021-06-16"
headers["Authorization"] = "Bearer ACCESS TOKEN"
headers["Content-Type"] = "application/json"
resp = requests.get(url, headers=headers)
print(resp.status_code)
decoded = jsonpickle.decode(resp.text)
Another example of a non-trivial API call can be seen here, pulled from the User Authentication documentation for the Dropbox API:
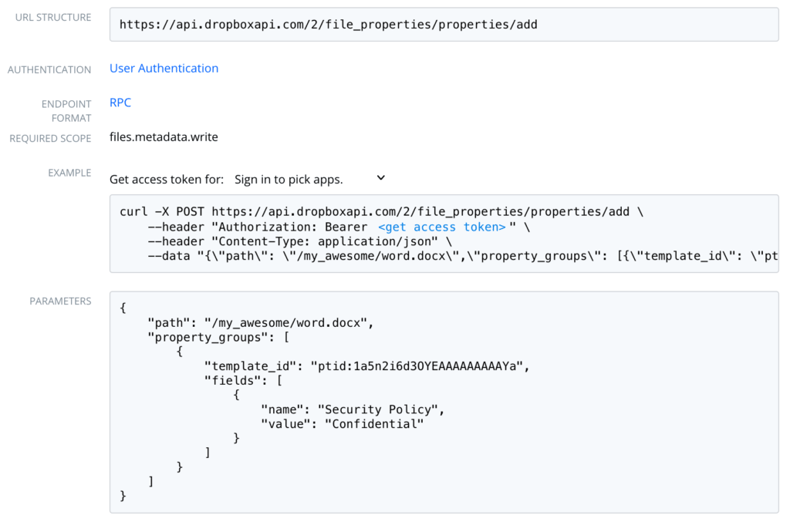
For many developers, the need to specify every parameter is exactly what they want: the full power of the service at their fingertips.
Low-level control can be unnecessary and overwhelming, though, especially for new developers. With just the basic building blocks, it can become a challenge for developers to perform more complicated tasks.
Not only can direct API calls be complicated to do correctly, but they can also be frustrating to try to troubleshoot. API response codes aren't particularly specific when providing error messages; Many developers may give up after countless generic 404 errors.
Ultimately, many developers will wish they could have something a little more prepared for them. For those developers, you can please them by offering SDKs in their language.
SDKs: What Are They?
SDKs are a compilation of environment-specific developer tools designed to access your API. SDKs make it more straightforward for developers to use your API using a programming language framework they prefer. For the developer who wants a little more guidance, SDKs will be the most appealing way for them to access your service.
SDKs abstract away the minutiae of the API that often bog down implementation for developers. They can be thought of as an API wrapper that makes accessing that API more friendly to a particular type of developer. A developer has to download and install an SDK that matches the language of their development environment, where it will then provide a host of streamlined functionalities for interacting with your API. If the developer is using Python, for instance, they’ll most likely download an SDK through pip, such as this example from Algolia’s documentation:
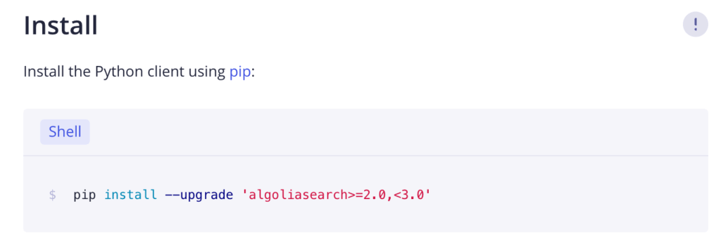
The example call to Dropbox's API, shown earlier, constructed with their Python SDK is shown below. In contrast to a direct API call, it does not require the developer to hard code a URL and headers. Instead, the API token is passed to a provided client object that handles those details.
import dropbox
token = 'My-API-Token'
dbx = dropbox.Dropbox(token)
My_Field = dropbox.file_properties.PropertyField('Security Policy','Confidential')
My_Template_ID = 'ptid:1...'
My_Property_Group = dropbox.file_properties.PropertyGroup(template_id=My_Template_ID, fields=[My_Field])
dbx.file_properties_properties_add("/my_awesome/word.docx", My_Property_Group)
While APIs aren't language-specific, SDKs are. A developer will download the SDK for the specific language they want to work with. API providers that intend to offer SDKs have to research what languages most appeal to their core user base. Developers who have the use of an SDK will be incredibly satisfied by the bevy of tools made available for them, including, but not limited to:
- Autocomplete SDK commands inline
- Code samples for commands
- Example applications
A beneficial everyday use case where SDKs are much easier to use than direct API calls is when developers need to perform complicated authentication procedures. Doing this correctly and securely with only direct API calls can be tricky, but most SDKs take these steps and make them easily implementable with just a few commands. The low-level API call parameters and structure are abstracted away from the developer and handled by the SDK. In many ways, SDKs are the epitome of one of the main purposes of APIs: to give the developer the ability to build off existing technology as easily and quickly as possible.
For many developers, SDKs are the preferred method of interacting with APIs. For others, there are cases for making direct API calls without an SDK. Now that we've looked at both options let's see why developers would lean towards one or the other.
Choosing Between SDKs and Direct API Calls
In an ideal world, there will be an SDK available to meet the developer’s exact needs. SDKs cost resources to produce and maintain, however. And even when SDKs are available, there are quite a few situations where the available SDKs might not work for what a developer wants to do. Just to list a few:- An SDK may not be available in their desired language
- The SDK may not be updated to incorporate new API features yet
In both of these situations, directly using the API might be the only option. After all, it takes time and effort for an API provider to build SDKs. If there's only one Julia developer using your API who wants a Julia SDK, it might not justify the use of resources.
Even for the SDKs of languages you deem popular enough to support, it takes time and effort to update those SDKs. Functionality added to an API yesterday may not be supported in the SDK by today.
For the vast majority of use cases, developers will appreciate having SDKs available and will eagerly use them. This is especially true for those who are making use of multiple APIs in their applications. For that reason, SDKs are worth the time and effort to develop for your primary user groups. The effort it takes to provide up-to-date SDKs will save them effort as they can seamlessly incorporate API calls into their app code.
And for those edge case developers, those who code in esoteric languages, those who need maximum security control over what the API is doing, and those who want to be on the cutting edge no matter what, direct API calls are most useful.
A great example of a company showing both direct API and SDK calls in their documentation is Stripe:
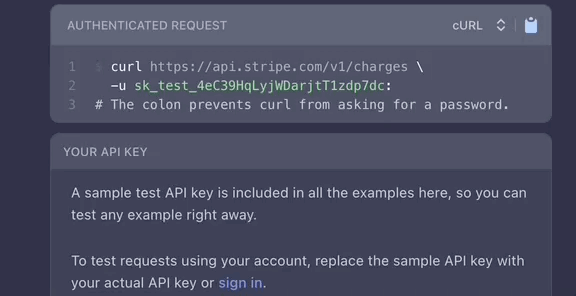
Both direct API calls and SDKs are tools that you should make available in your developers' toolboxes. And the more tools at their disposal, the more effective your developers will be.
At the end of the day, the goal of your developer experience should be improving the accessibility of your product to developers of all backgrounds. Providing SDKs is the best way to make your API a little more manageable for them to implement.
Have a look at how APIMatic enhances the developer experience of your API with robust SDKs auto-generated in all popular languages from a single API definition file.